Debug Kotlin/Wasm code
This tutorial demonstrates how to use your browser to debug your Compose Multiplatform application built with Kotlin/Wasm.
Before you start
Create a project using the Kotlin Multiplatform wizard:
Open the Kotlin Multiplatform wizard.
On the New Project tab, change the project name and ID to your preference. In this tutorial, we set the name to "WasmDemo" and the ID to "wasm.project.demo".
Select the Web option. Make sure that no other options are selected.
Click the Download button and unpack the resulting archive.
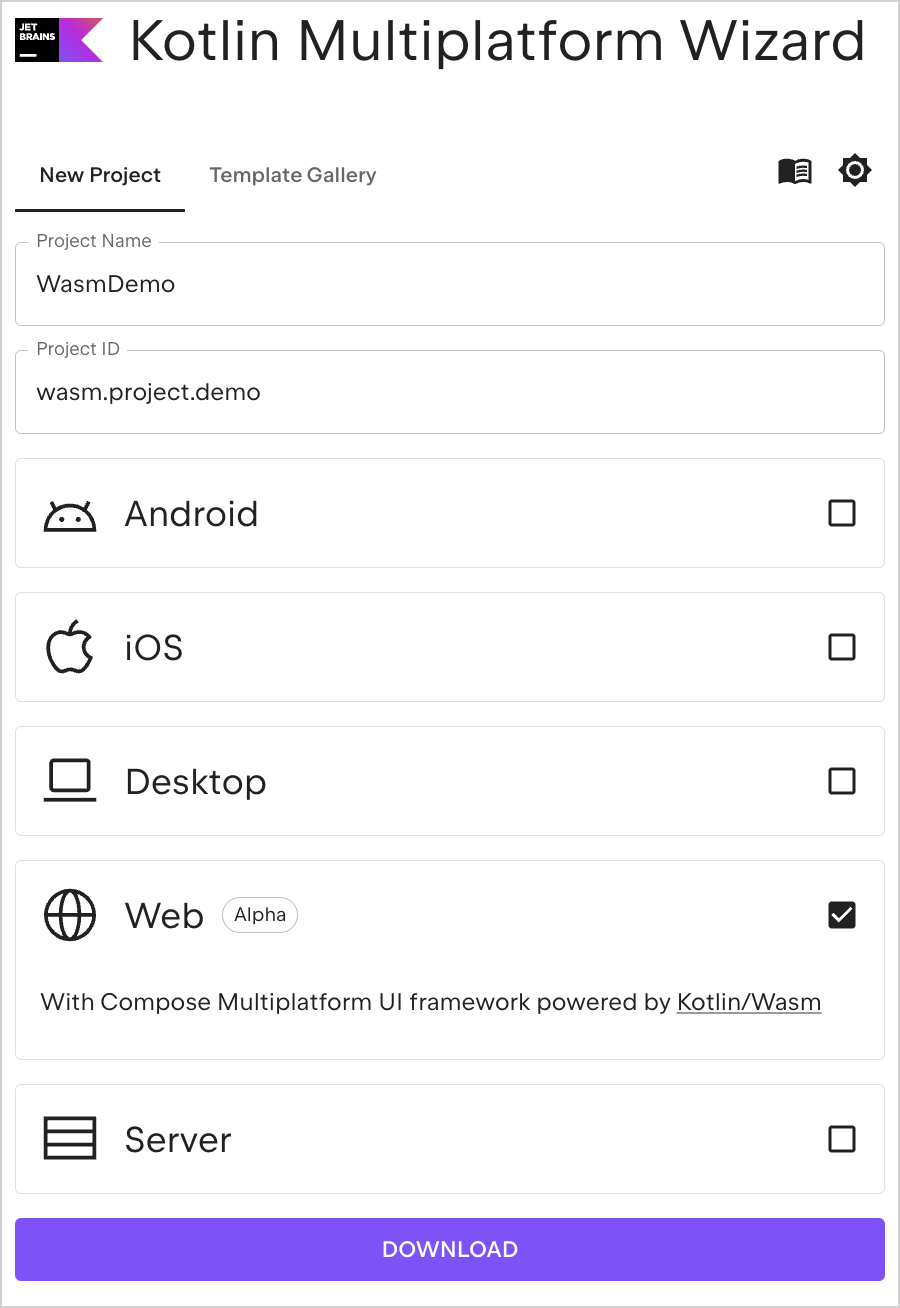
Open the project in IntelliJ IDEA
Download and install the latest version of IntelliJ IDEA.
On the Welcome screen of IntelliJ IDEA, click Open or select File | Open in the menu bar.
Navigate to the unpacked "WasmDemo" folder and click Open.
Run the application
In IntelliJ IDEA, open the Gradle tool window by selecting View | Tool Windows | Gradle.
In composeApp | Tasks | kotlin browser, select and run the wasmJsBrowserRun task.
Alternatively, you can run the following command in the terminal from the
WasmDemo
root directory:./gradlew wasmJsBrowserRunOnce the application starts, open the following URL in your browser:
http://localhost:8080/You see a "Click me!" button. Click it:
Now you see the Compose Multiplatform logo:
Debug in your browser
You can debug this Compose Multiplatform application in your browser out of the box, without additional configurations.
However, for other projects, you may need to configure additional settings in your Gradle build file. For more information about how to configure your browser for debugging, expand the next section.
Configure your browser for debugging
Enable access to project's sources
By default, browsers can't access some of the project's sources necessary for debugging. To provide access, you can configure the Webpack DevServer to serve these sources. In the ComposeApp
directory, add the following code snippets to your build.gradle.kts
file.
Add this import as a top-level declaration:
Add this code snippet inside the commonWebpackConfig{}
block, located in the wasmJs{}
target DSL and browser{}
platform DSL within kotlin{}
:
The resulting code block looks like this:
Enhance your debugging with custom formatters
In addition to the default debugging configuration, you can set up custom formatters to display and locate variable values in a more user-friendly and comprehensible manner.
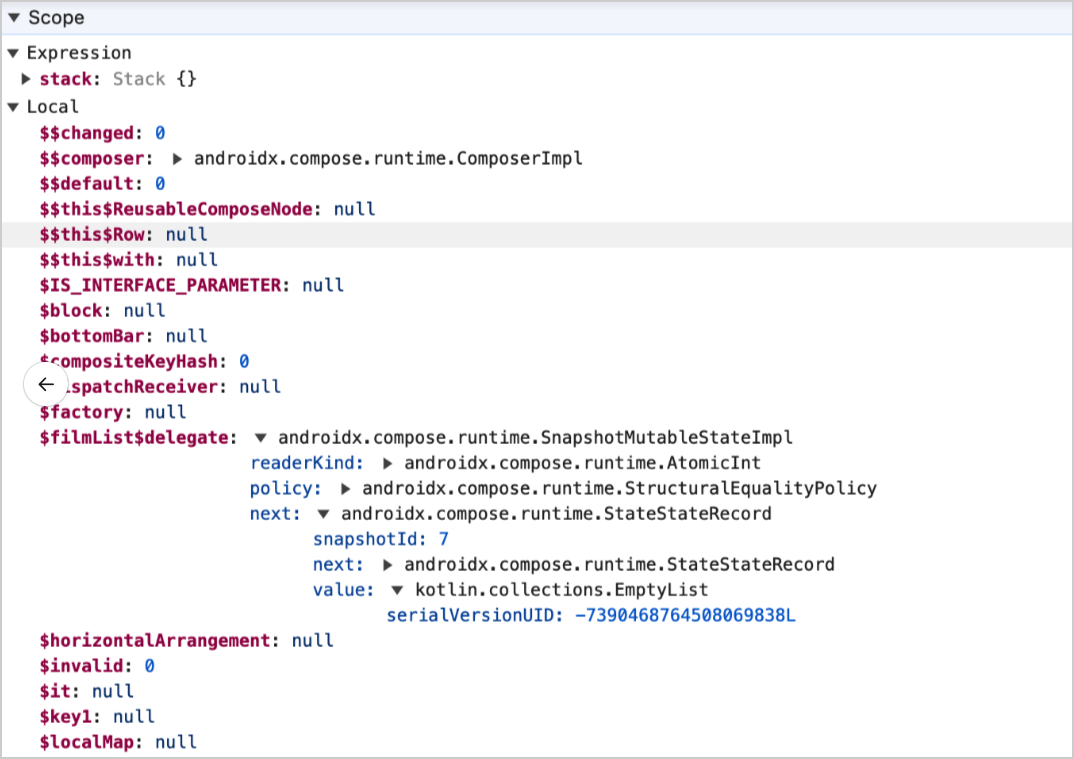
This implementation is supported across major browsers like Firefox and Chromium-based as it uses the custom formatters API.
To set up custom formatters for an improved debugging experience:
Add the following compiler argument to the
wasmJs
compiler options:kotlin { wasmJs { // ... compilerOptions { freeCompilerArgs.add("-Xwasm-debugger-custom-formatters") } } }Enable the Custom formatters feature in your browser:
In the Chrome DevTools, it's placed in Settings | Preferences | Console:
In the Firefox Developer Tools, it's placed in Settings | Advanced settings:
After setting up custom formatters, you can complete the debugging tutorial.
Debug your Kotlin/Wasm application
In the browser window of the application, right-click and select the Inspect action to access developer tools. Alternatively, you can use the F12 shortcut or select View | Developer | Developer Tools.
Switch to the Sources tab and select the Kotlin file to debug. In this tutorial, we'll work with the
Greeting.kt
file.Click on the line numbers to set breakpoints on the code that you want to inspect. Only the lines with darker numbers can have breakpoints.
Click on the Click me! button to interact with the application. This action triggers the execution of the code, and the debugger pauses when the execution reaches a breakpoint.
In the debugging pane, use the debugging control buttons to inspect variables and code execution at the breakpoints:
Step into to investigate a function more deeply.
Step over to execute the current line and pause on the next line.
Step out to execute the code until it exits the current function.
Check the Call stack and Scope panes to trace the sequence of function calls and pinpoint the location of any errors.
For an improved visualization of the variable values, see Enhance your debugging with custom formatters.
Make changes to your code and run the application again to verify that everything works as expected.
Click on the line numbers with breakpoints to remove the breakpoints.
Leave feedback
We would appreciate any feedback you may have on your debugging experience!
Slack: get a Slack invite and provide your feedback directly to the developers in our #webassembly channel.
Provide your feedback in YouTrack.
What's next?
See Kotlin/Wasm debugging in action in this YouTube video.
Try the Kotlin/Wasm examples from our
kotlin-wasm-examples
repository: