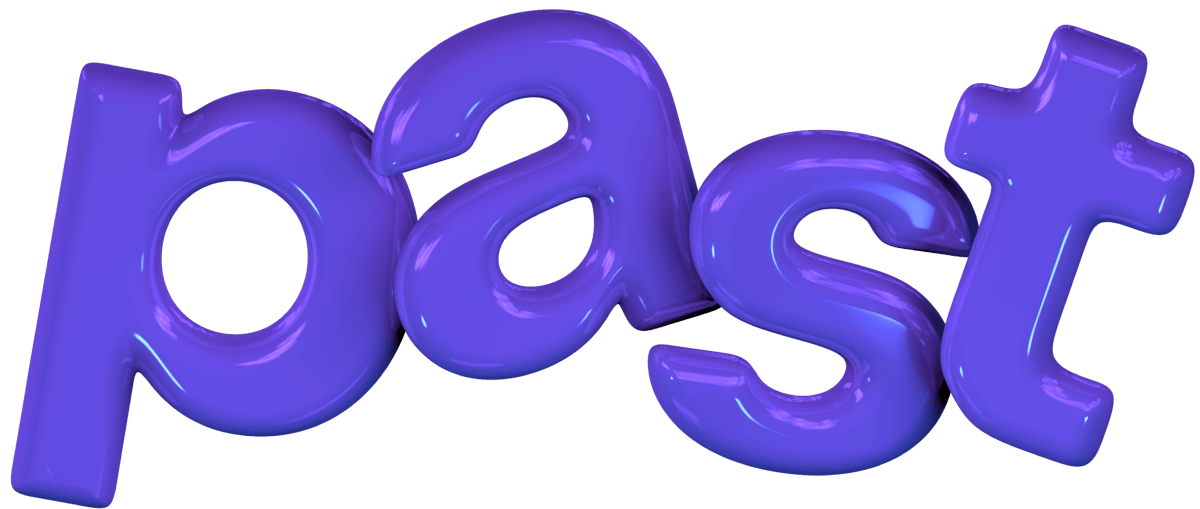
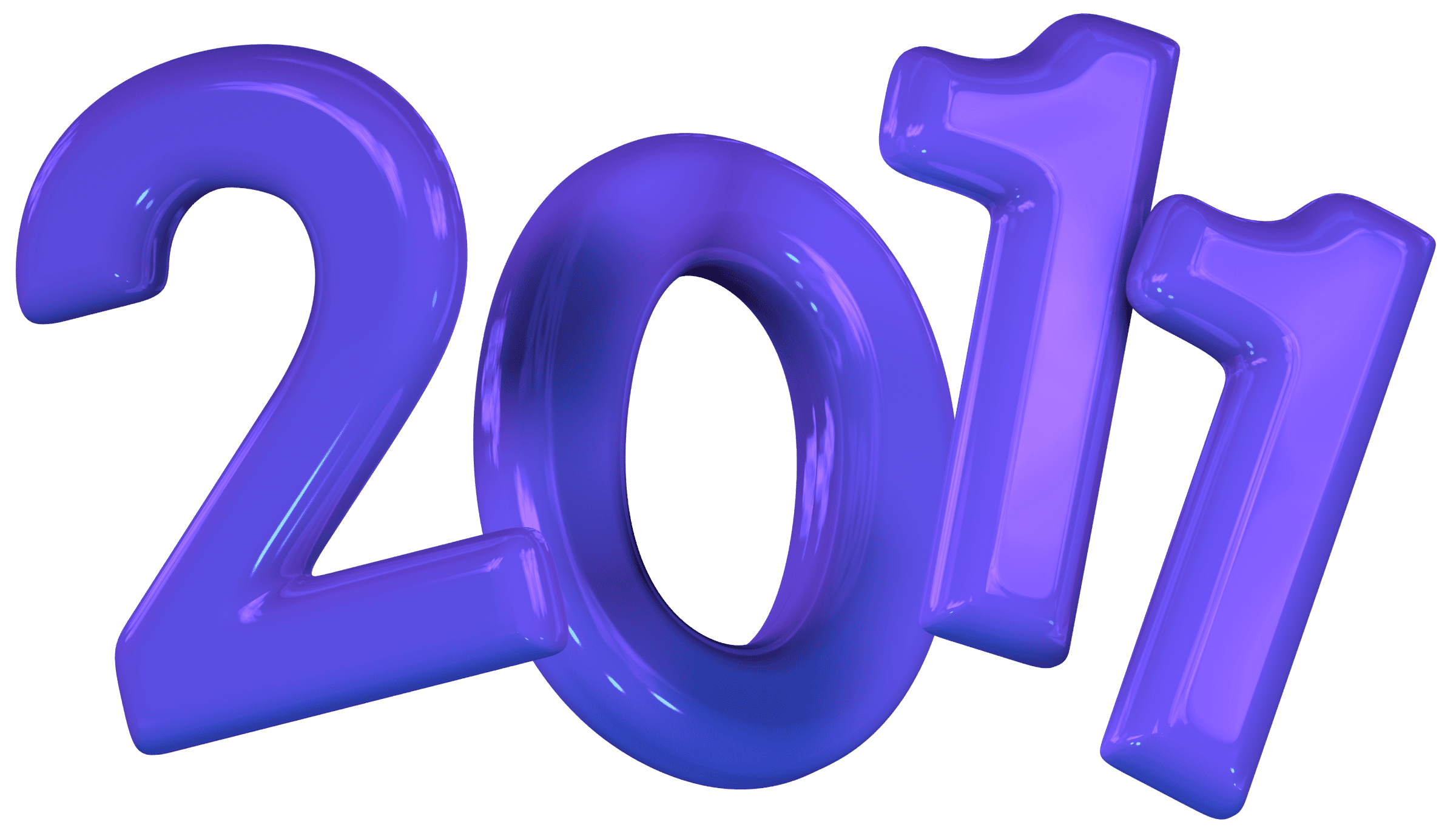
Hello world
The first syntax prototype
Function types and literals
//Functions
fun f(p: Int) : String { return p.toString() }
//Function types
fun (p: Int) : String
fun (Int) : String
//Function literals
{ (p: Int) : String => p.toString()} {(p : Int) => p.toString() }
{p => p.toString()}
Higher-order functions
fun filter<T>(
c: Iterable<T>,
f: fun (T) : Boolean
): Iterable<T>
val list = list("a", "ab", "abc", "") filter(list, { s => s.length() < 3 })
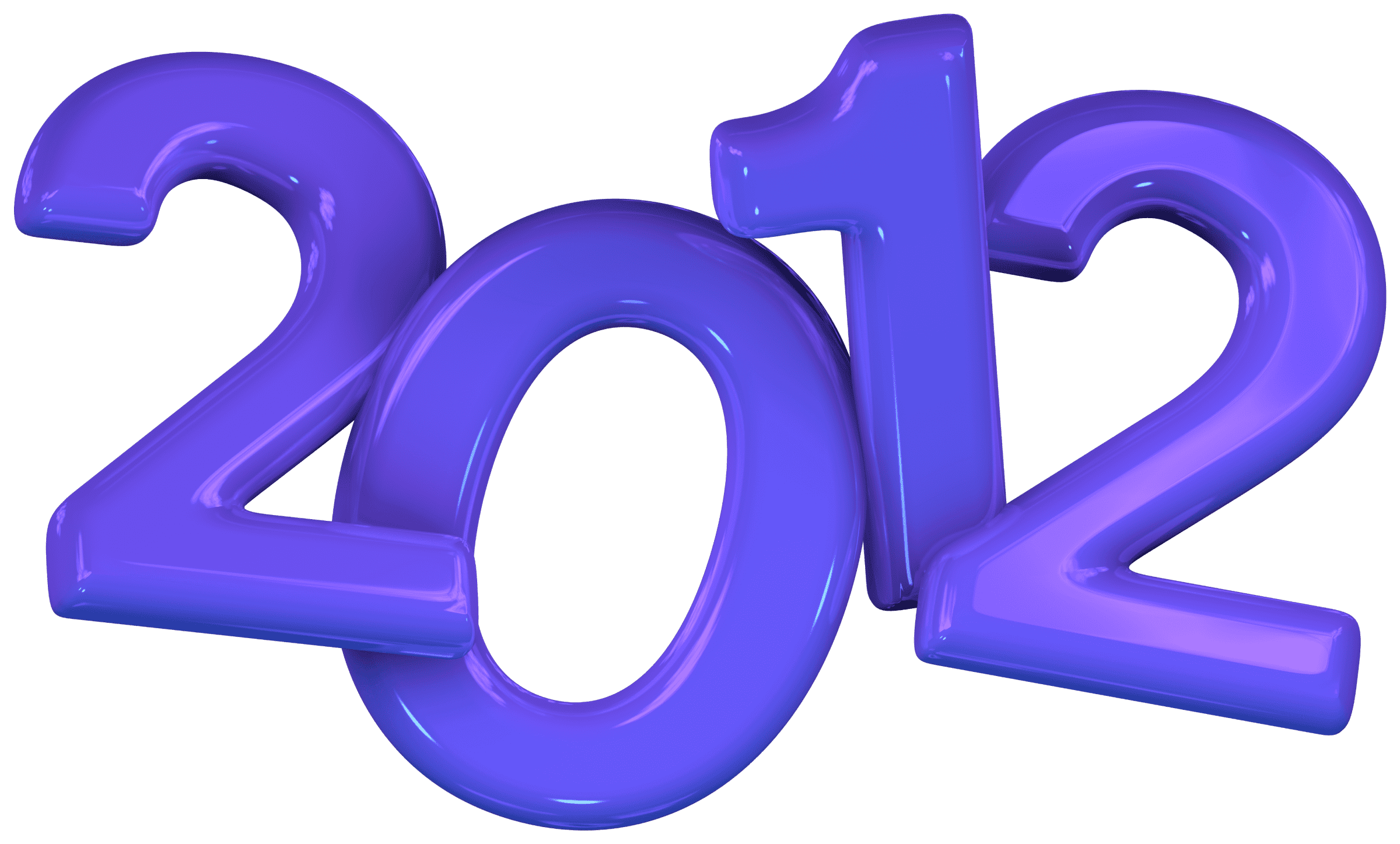
its first update
The Great Syntactic Shift:
- Namespace died: the namespace keyword was replaced by package.
- The Arrow lost weight: now we use a “thin arrow” (->) instead of a “thick arrow” (=>).
- Function types became more readable:
// before:
fun max(col: Collection<Int>, compare: fun(Int, Int): Int): Int
// after:
fun max(col: Collection<Int>, compare: (Int, Int) -> Int): Int
The first Kotlin web demo
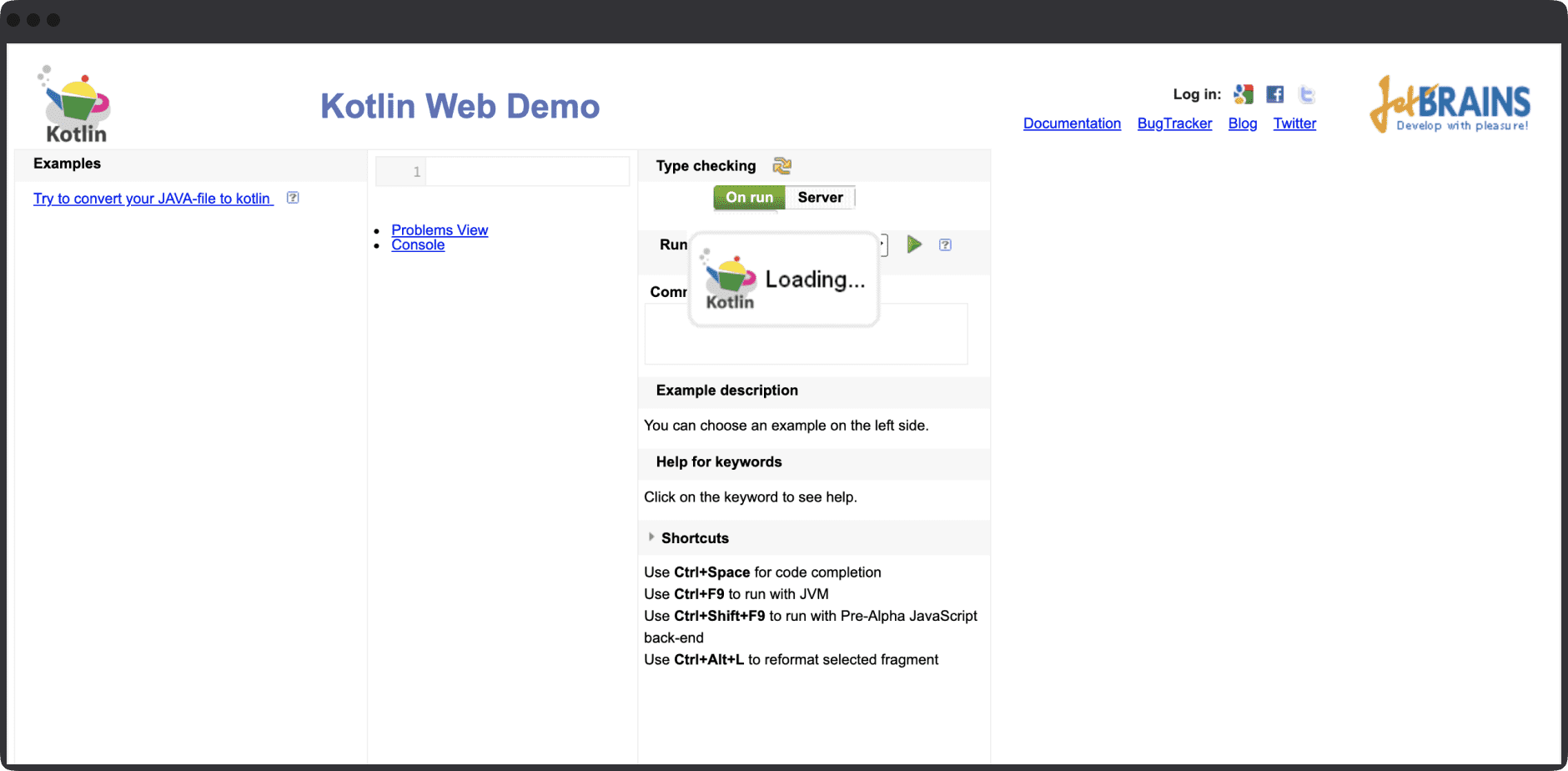
We went open source!
Kotlin started running on Android
package com.example
import android.app.Activity
import android.os.Bundle
class HelloKotlin() : Activity() {
protected override fun onCreate(savedInstanceState: Bundle?) {
super<Activity>.onCreate(savedInstanceState)
setContentView(R.layout.main)
}
}
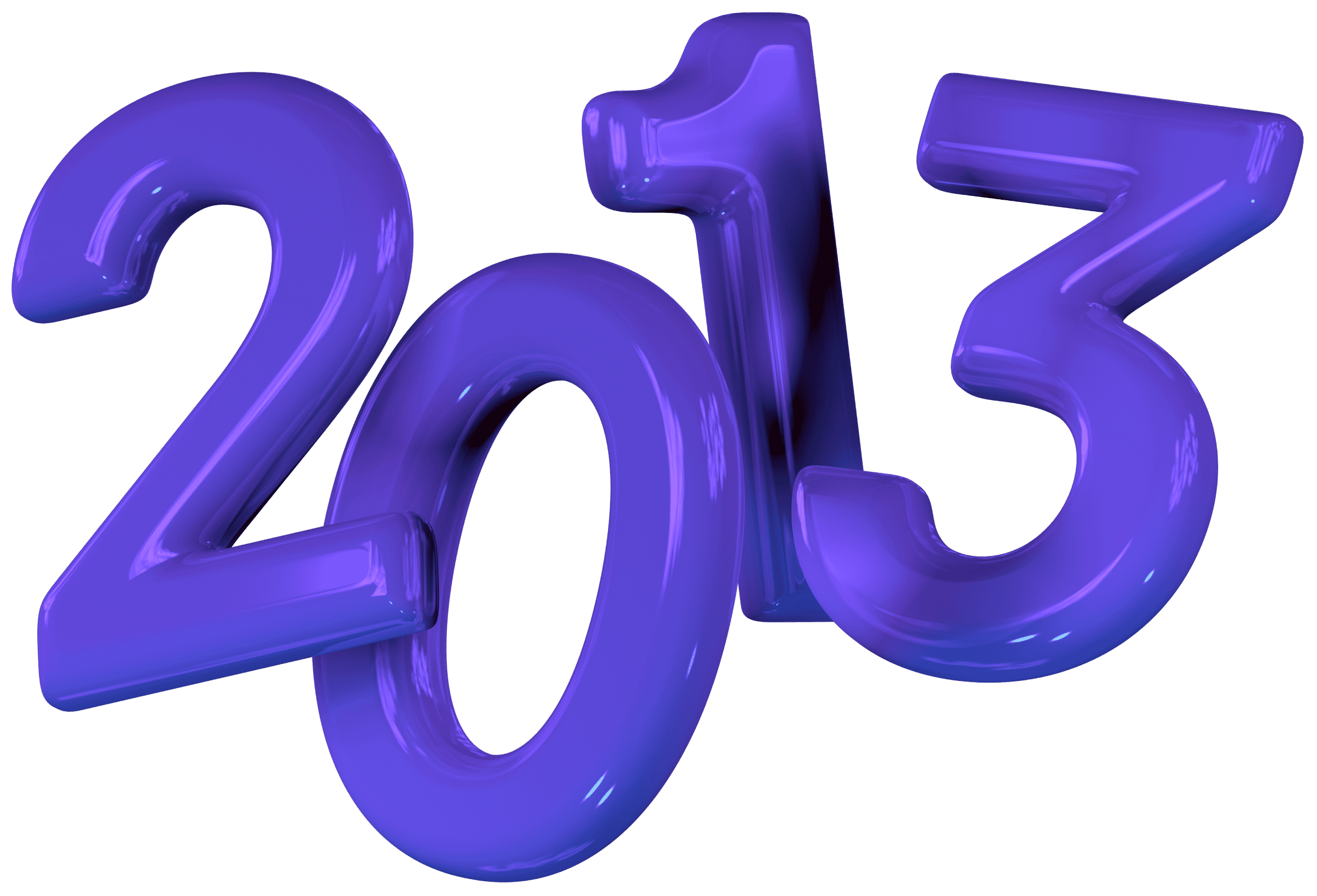
The first chunk of SAM-conversion
support appeared in Kotlin
New language features were added: delegated properties, callable references, static constants, and static fields
Delegated properties
class Delegate() {
fun get(thisRef: Any?, prop: PropertyMetadata): String {
return "$thisRef, thank you for delegating '${prop.name}' to me!"
}
fun set(thisRef: Any?, prop: PropertyMetadata, value: String) {
println("$value has been assigned")
}
}
Callable references
fun isOdd(x: Int) = x % 2 != 0
val numbers = listOf(1, 2, 3)
println(numbers.filter(::isOdd)) // Prints [1, 3]
The Gradle
plugin was released
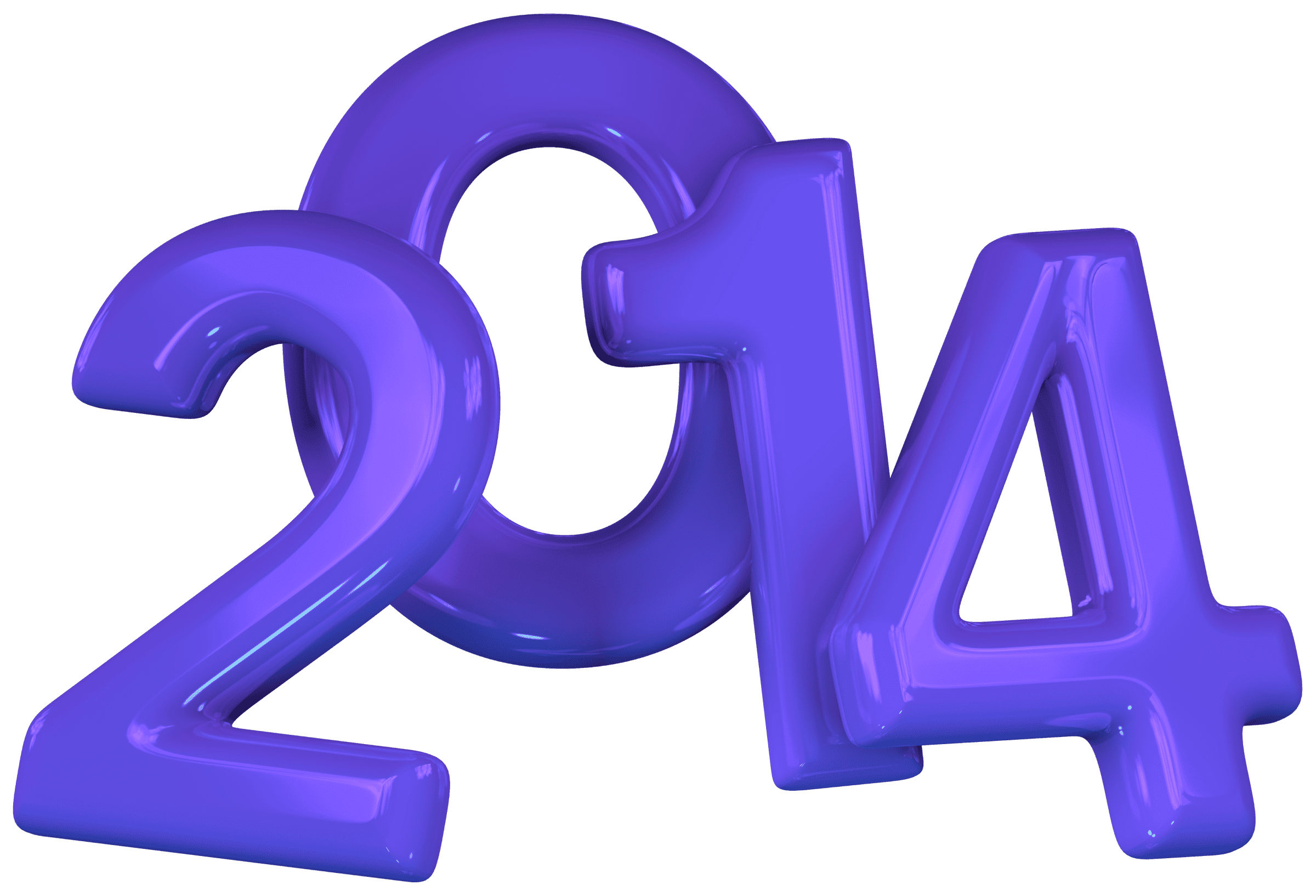
Kotlinlang.org was launched
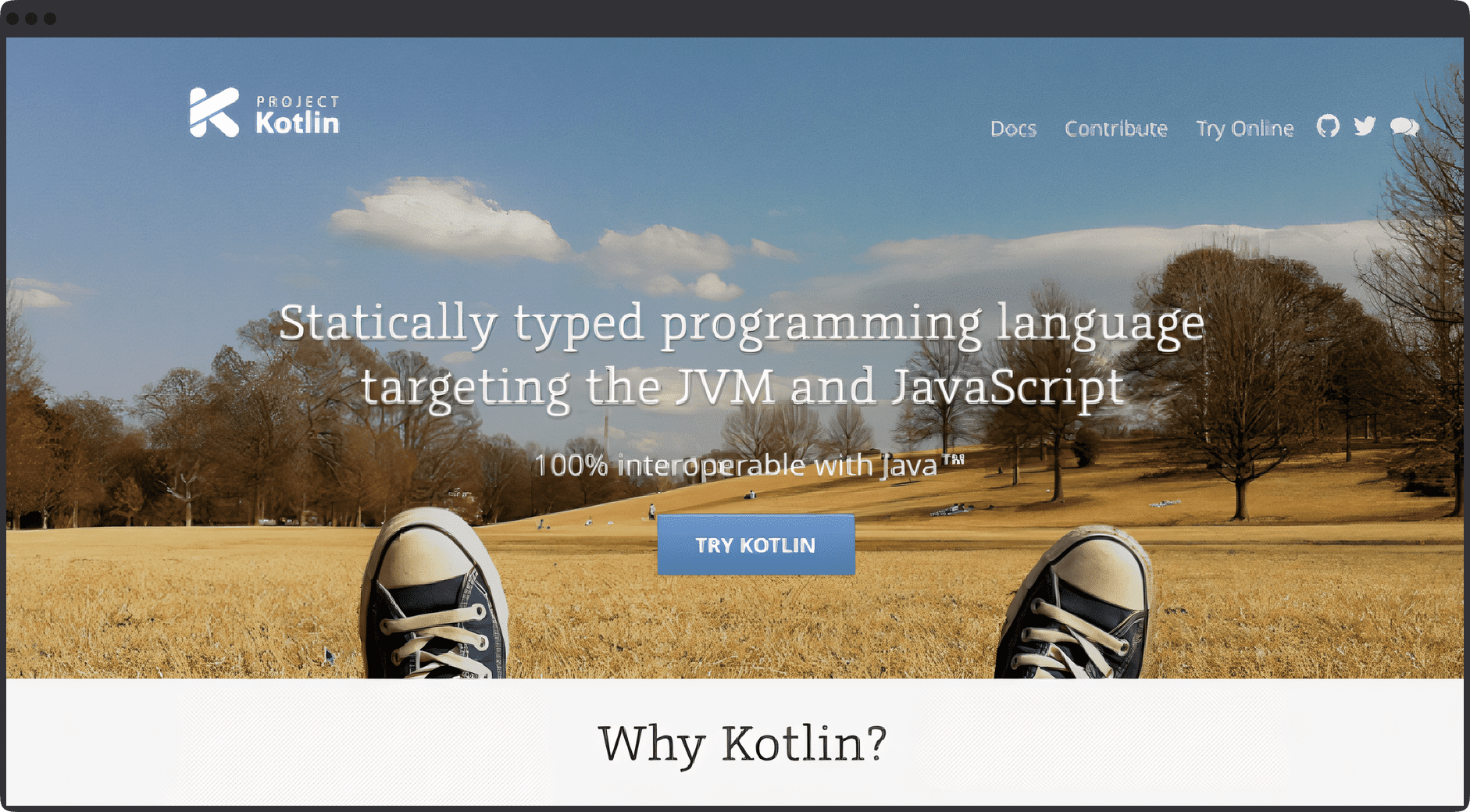
New features were added to improve JavaScript interop
Support for the dynamic keyword was added for declaring types as dynamic.
native("$")
val jquery : dynamic = noImpl
jquery.getJSON(KotlinCommitsURL) { commits: dynamic ->
val commitsTable = jquery("#kotlin-commits")
commits.forEach { commit: dynamic ->
commitsTable.append("""
${commit.sha.substring(0, 6)}
${commit.commit.message}
""")
}
}
It became possible to inline JavaScript code with the help of the js function.
jquery.getJSON(KotlinCommitsURL) { commits ->
js("console.log('Calling JavaScript')")
val commitsTable = jquery("#kotlin-commits")
}
Language Injection support for Kotlin was added to IntelliJ IDEA. This change enabled proper highlighting inside of the js function.
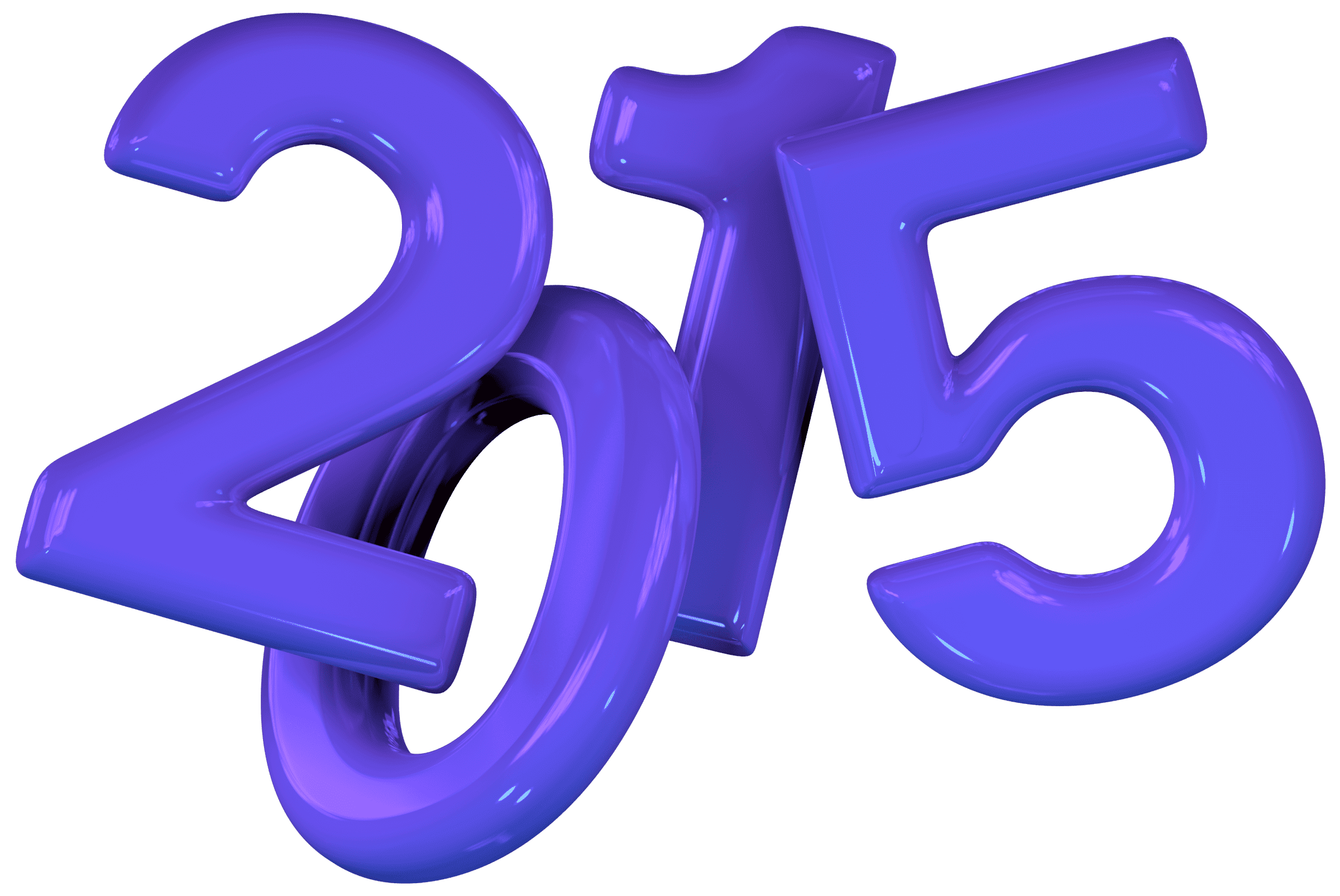
New features dramatically improved the developer experience on various platforms: multiple constructors, companion objects, sealed classes, lateinit properties
Multiple constructors
class MyView : View {
constructor(context: Context, attrs: AttributeSet, defStyle: Int): super(context, attrs, defStyle) {
// ...
}
constructor(context: Context, attrs: AttributeSet) : this(context, attrs, 0) {}
}
Before the 1.0 release, we took the opportunity to tidy the language up a bit:
- Removed all the deprecations that had accumulated as our libraries evolved.
- Removed all the deprecations from the generated code.
- Got rid of some legacy bytecode peculiarities that were found during the beta.
- Moved some of the stdlib code around to better structure the packages there.
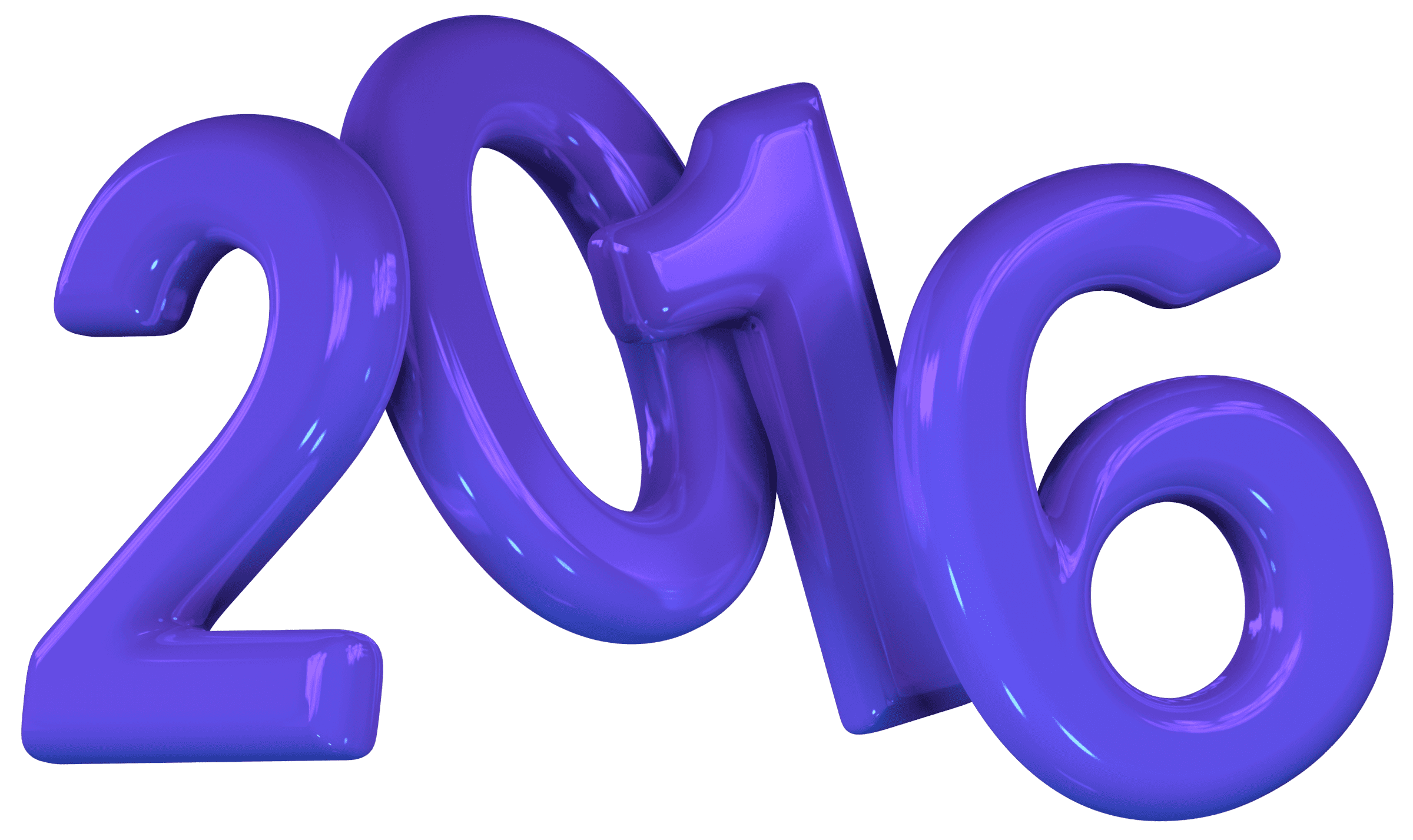
Kotlin 1.0 Released: Pragmatic Language for the JVM and Android 🎉
Gradle
met Kotlin
We demoed the first milestone of using Kotlin for Gradle build scripts.
Coroutines first appeared
Coroutines is a traditional CS term for “program components that generalize subroutines for non-preemptive multitasking”.
fun main(args: Array<String>) {
val future = async<String> {
(1..5).map {
await (startLongAsyncOperation(it)) // suspend while the long method is running
}.joinToString(" ")
}
println(future.get())
}
The first Kotlin Night took place in San Francisco
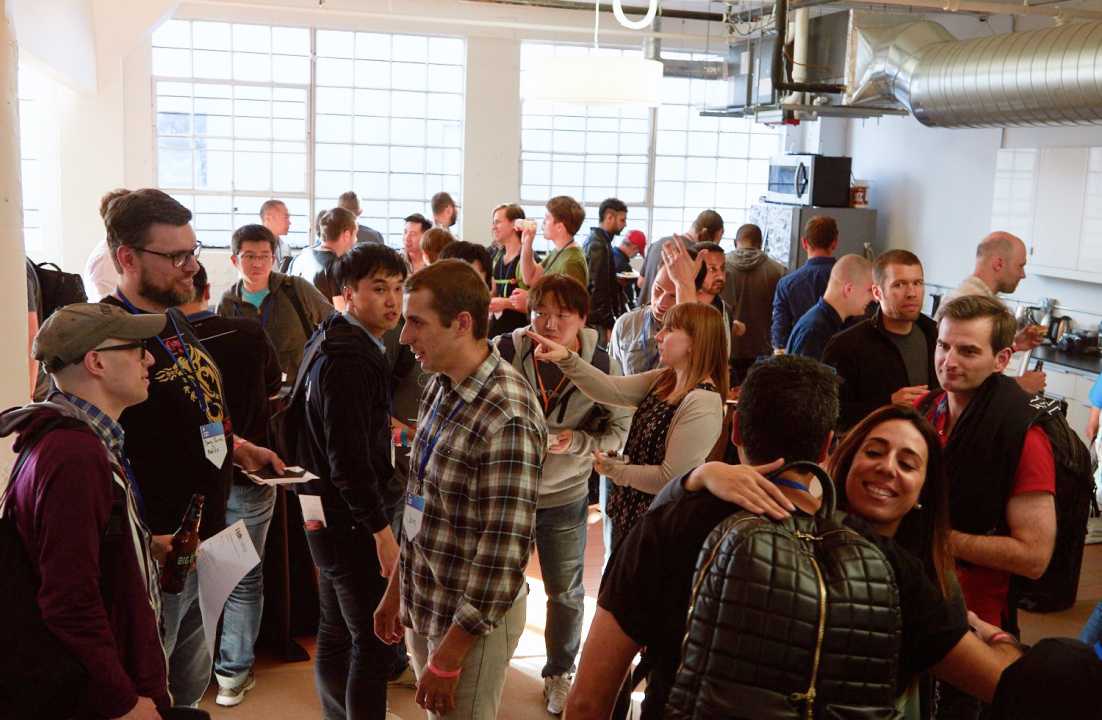
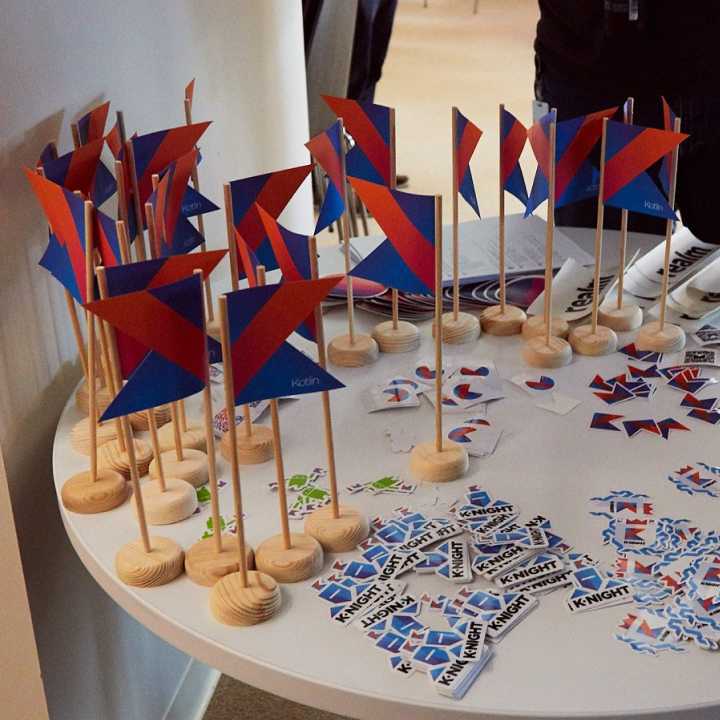
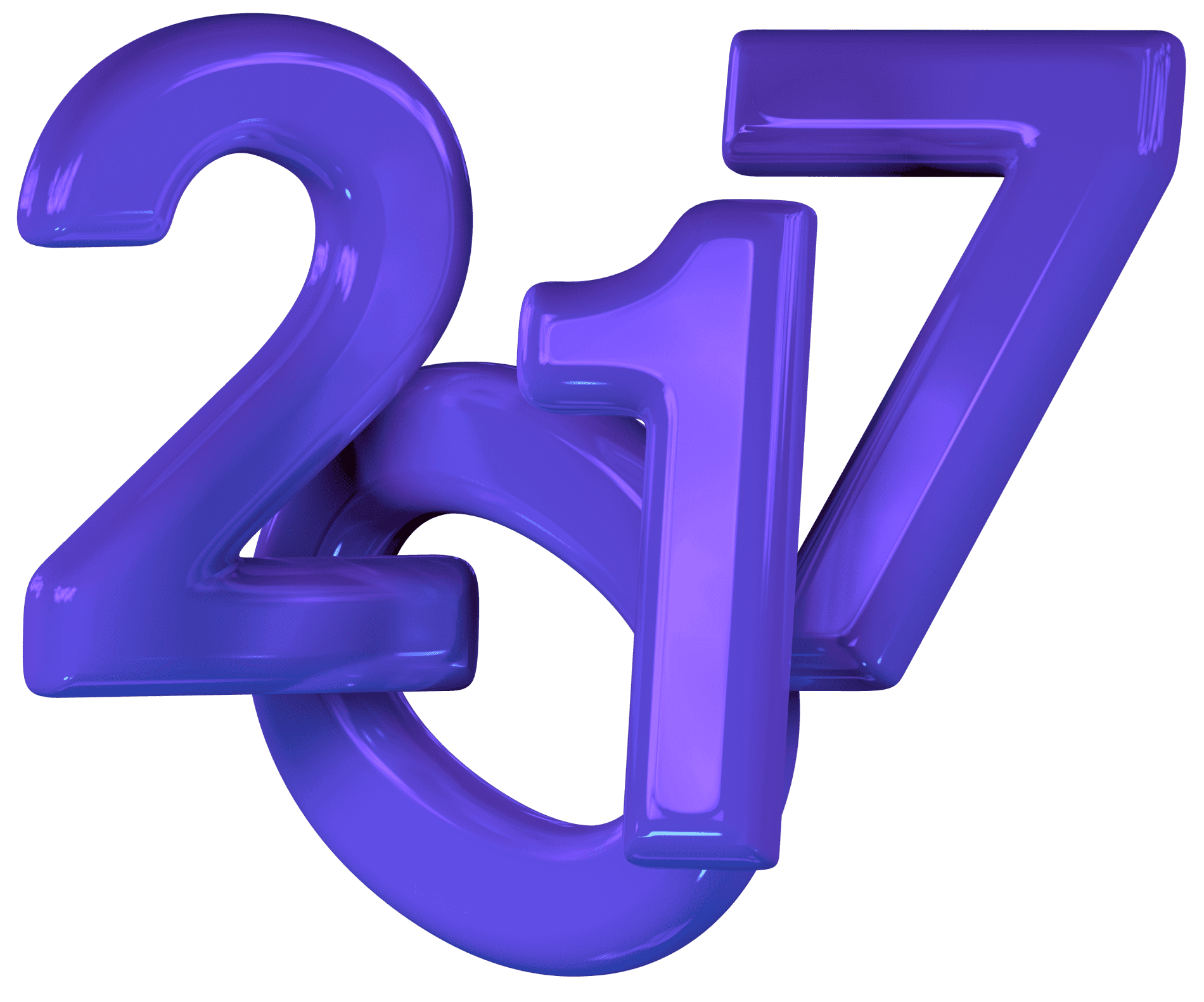
Kotlin on Android became official
At the Google I/O keynote, the Android team announced first-class support for Kotlin.
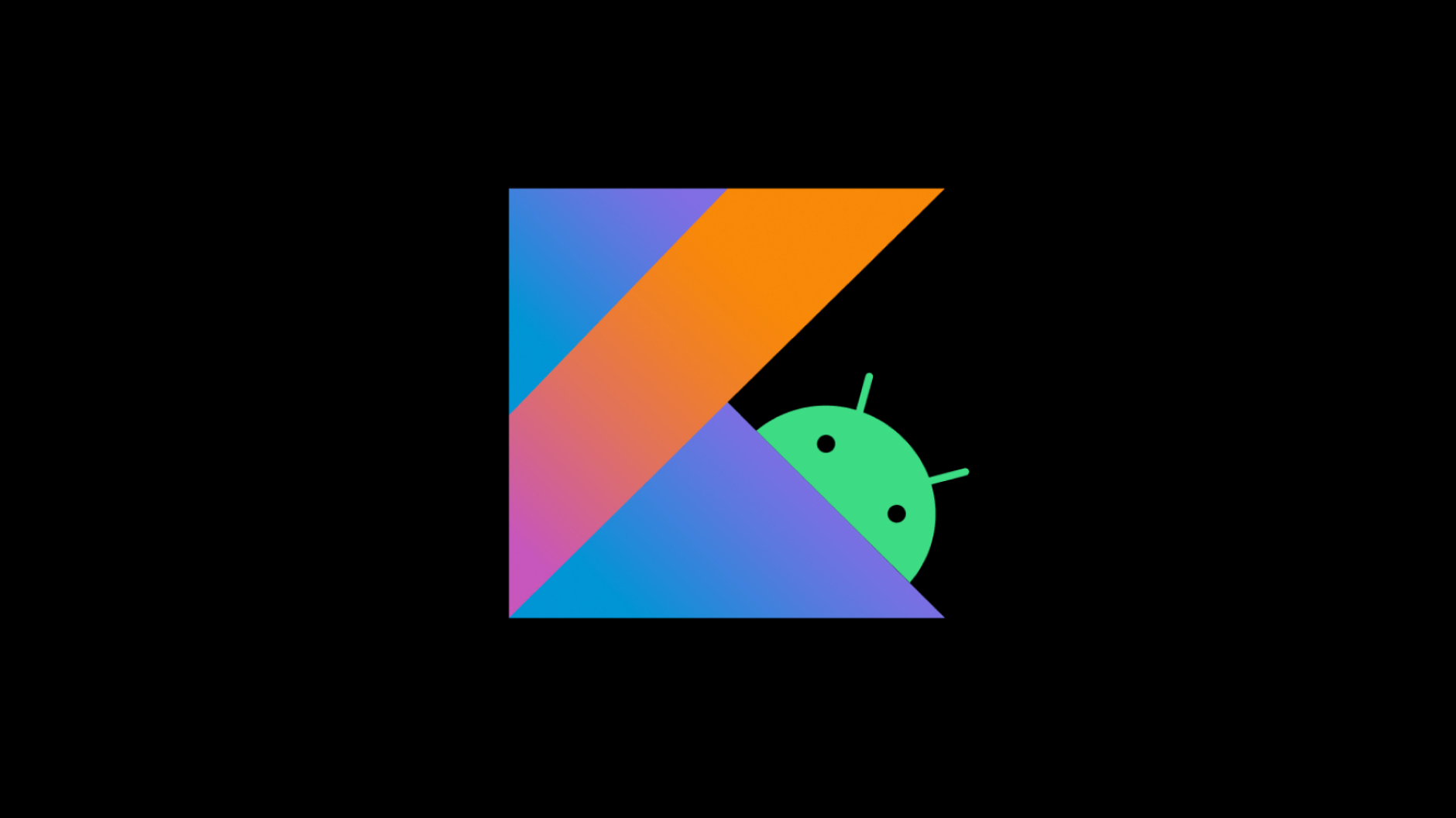
We launched our support program for Kotlin User Groups, and Kotlin Nights became a community-driven event series
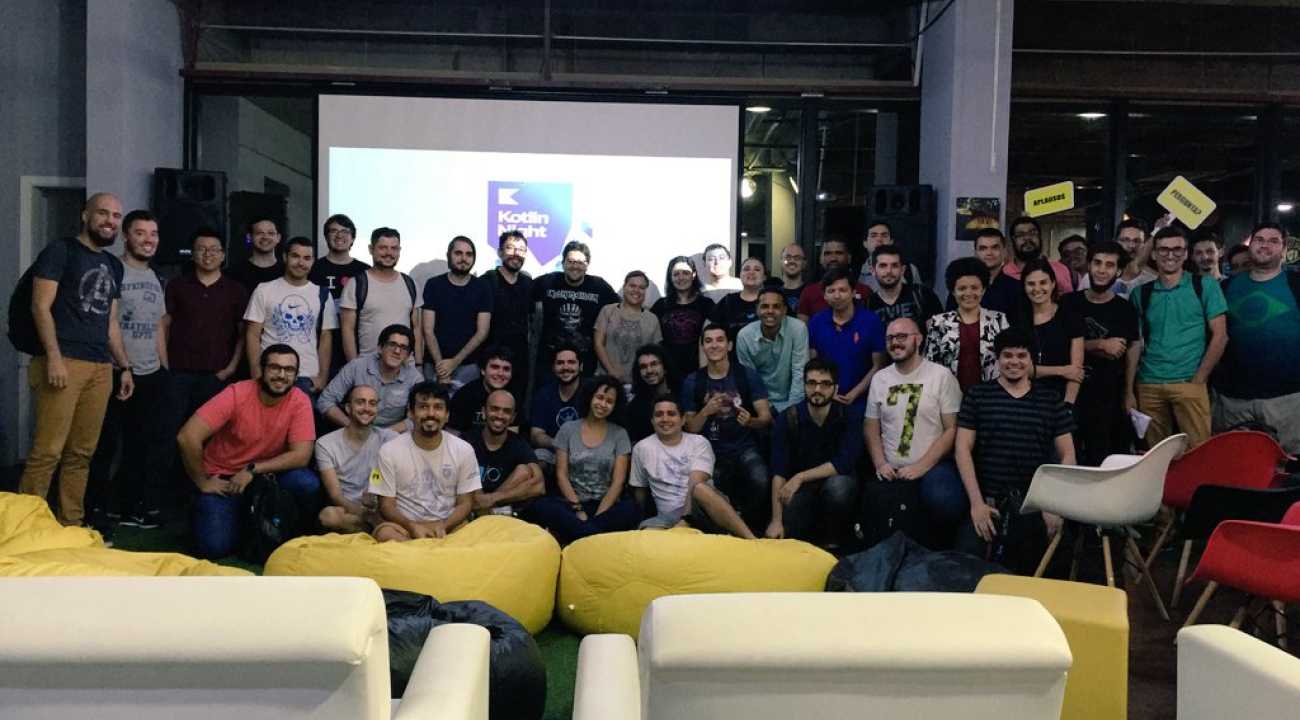
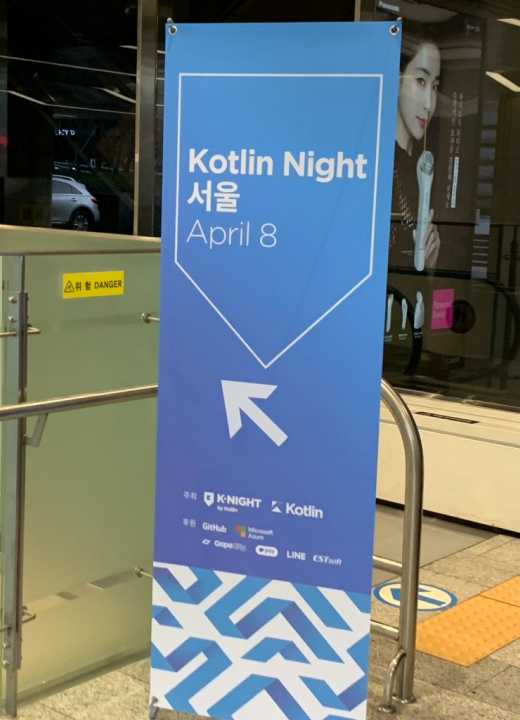
Our first book about Kotlin came out
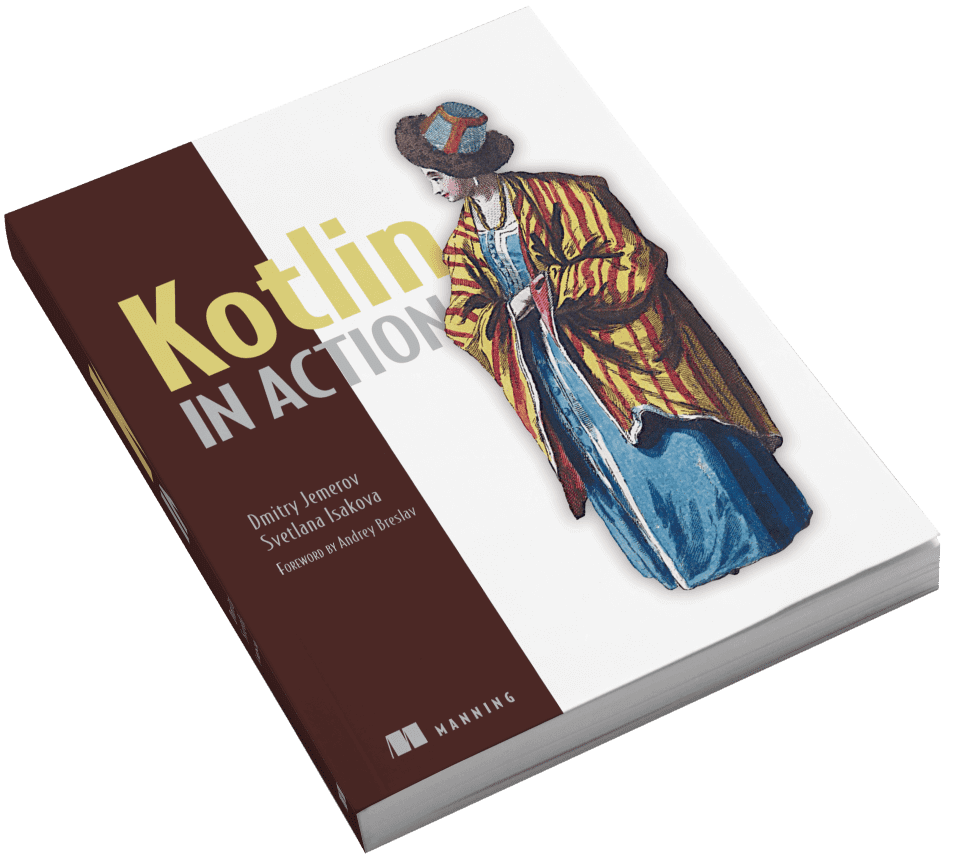
The first Technology Preview of Kotlin/Native appeared
This made it possible to run Kotlin without a virtual machine. Shortly after, code sharing became available.
The very first KotlinConf was held in San Francisco
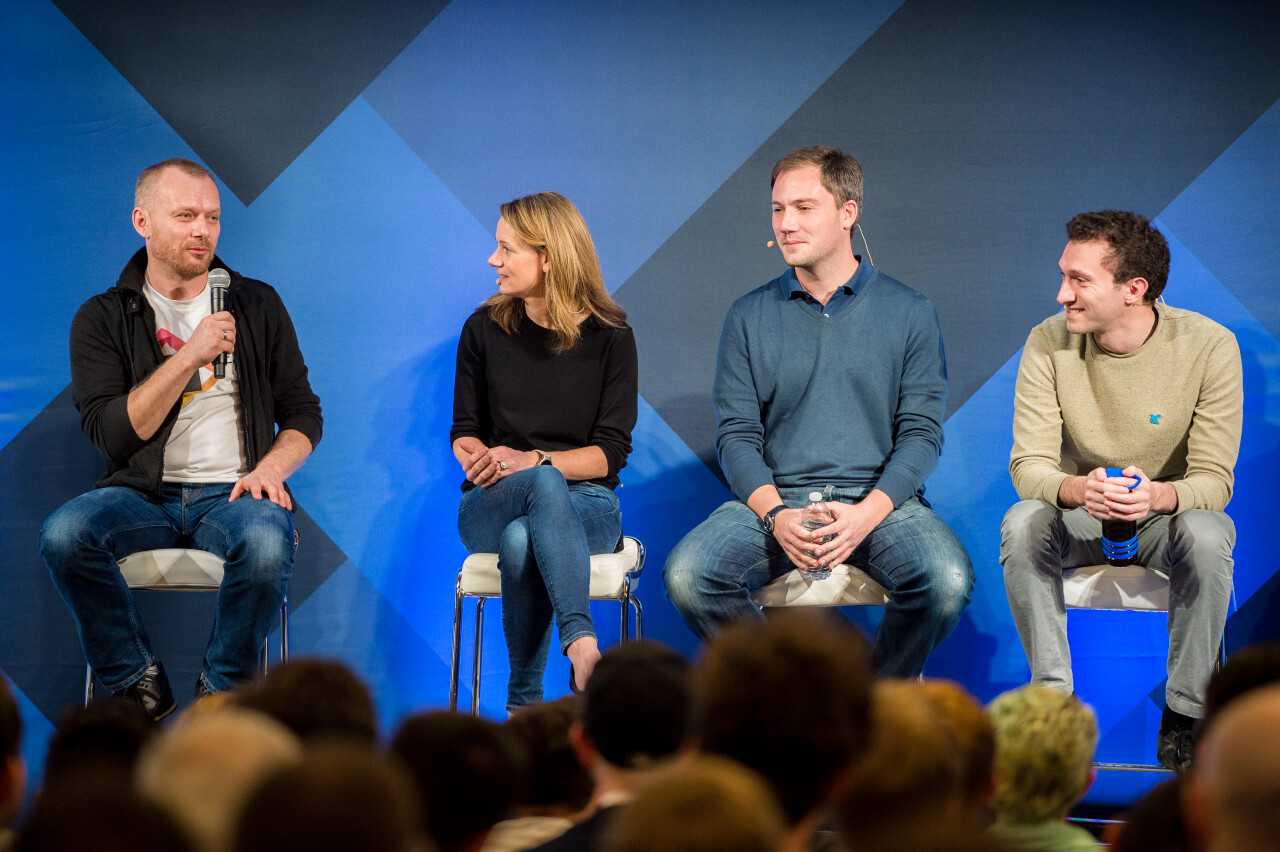
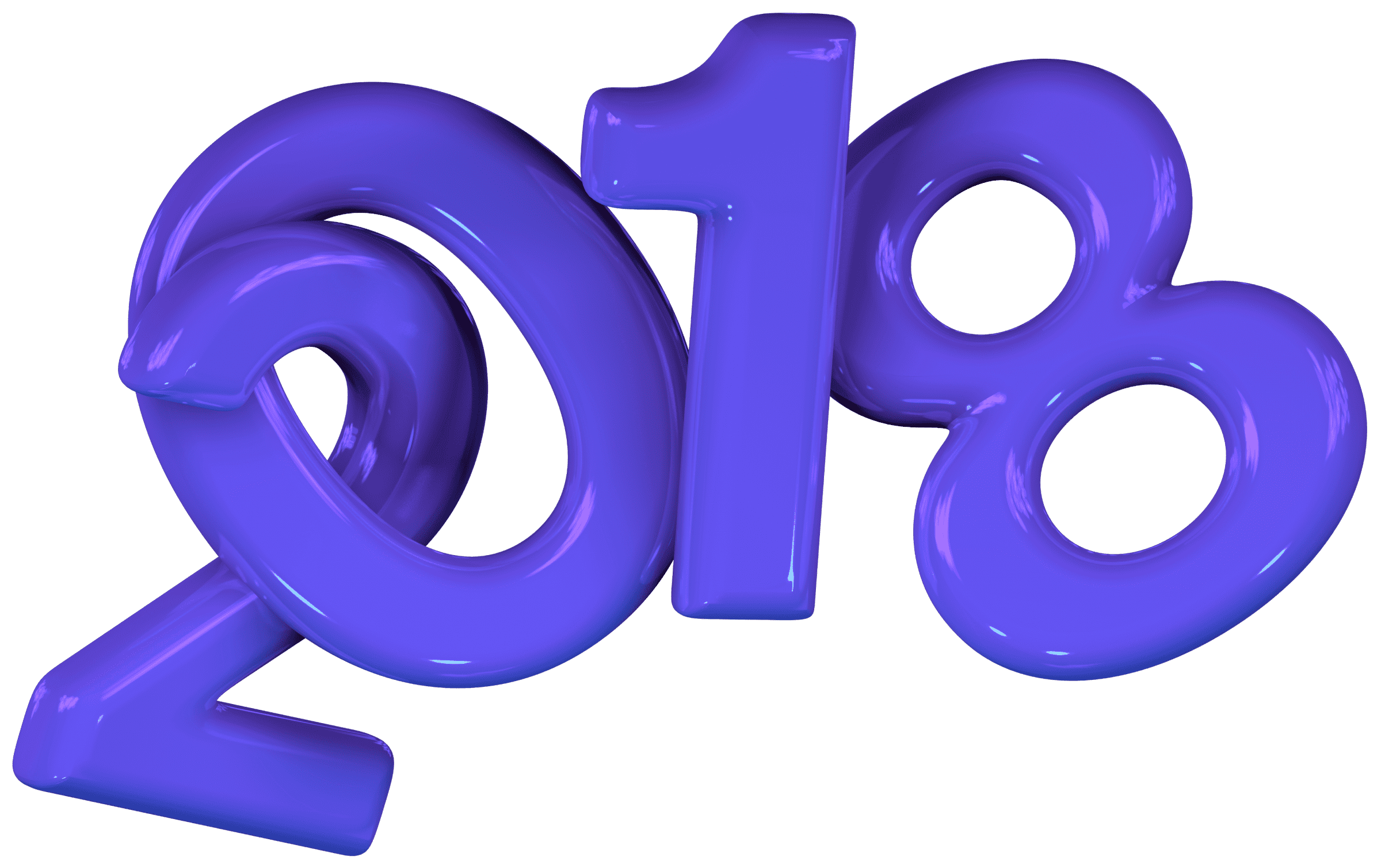
Ktor 1.0 was released
Ktor is a Kotlin framework for building asynchronous servers and clients in connected systems. It takes full advantage of the language in order to provide a great developer experience and excellent runtime performance.
fun main() {
embeddedServer(Netty, port = 8000) {
routing {
get ("/") {
call.respondText("Hello, world!")
}
}
}.start(wait = true)
}
Inline classes made it possible to wrap a value of a type without creating an actual wrapper object
inline class Name(internal val value: String)
We made it possible to embed runnable and editable Kotlin snippets into blog posts and other materials
Adding an embedded Kotlin playground is as easy as writing a single line in the page header.
<script src="https://unpkg.com/kotlin-playground@1" data-selector="code"></script>
KotlinConf 2018 was held in Amsterdam
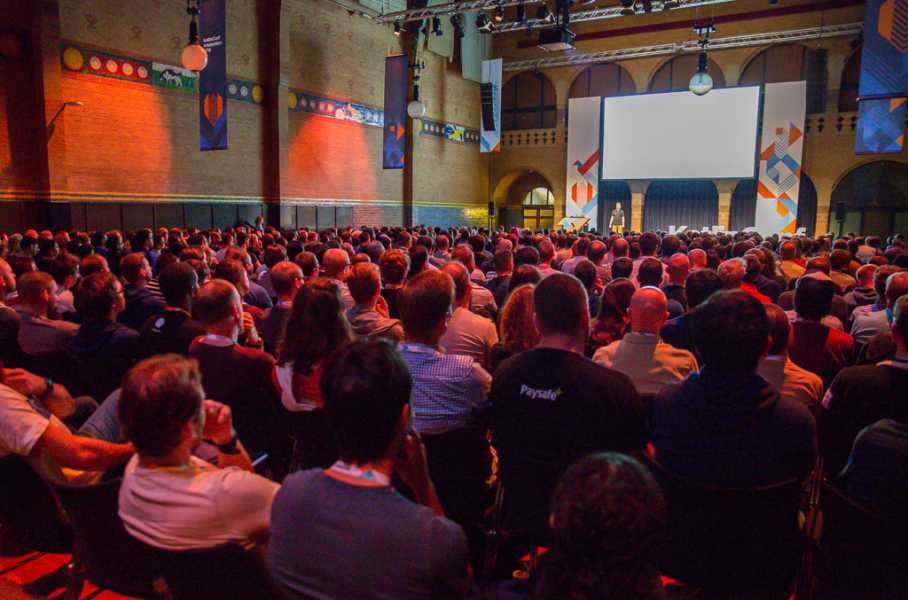
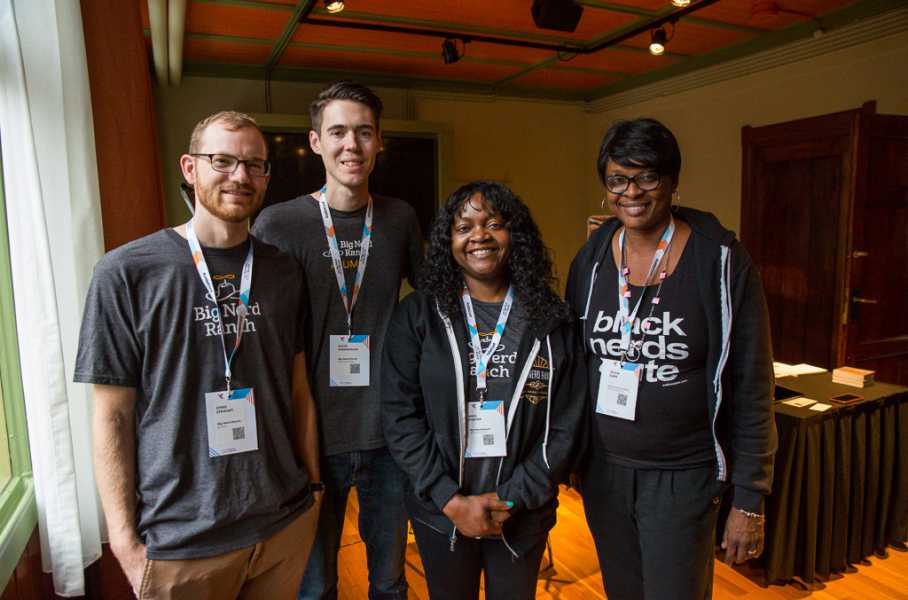
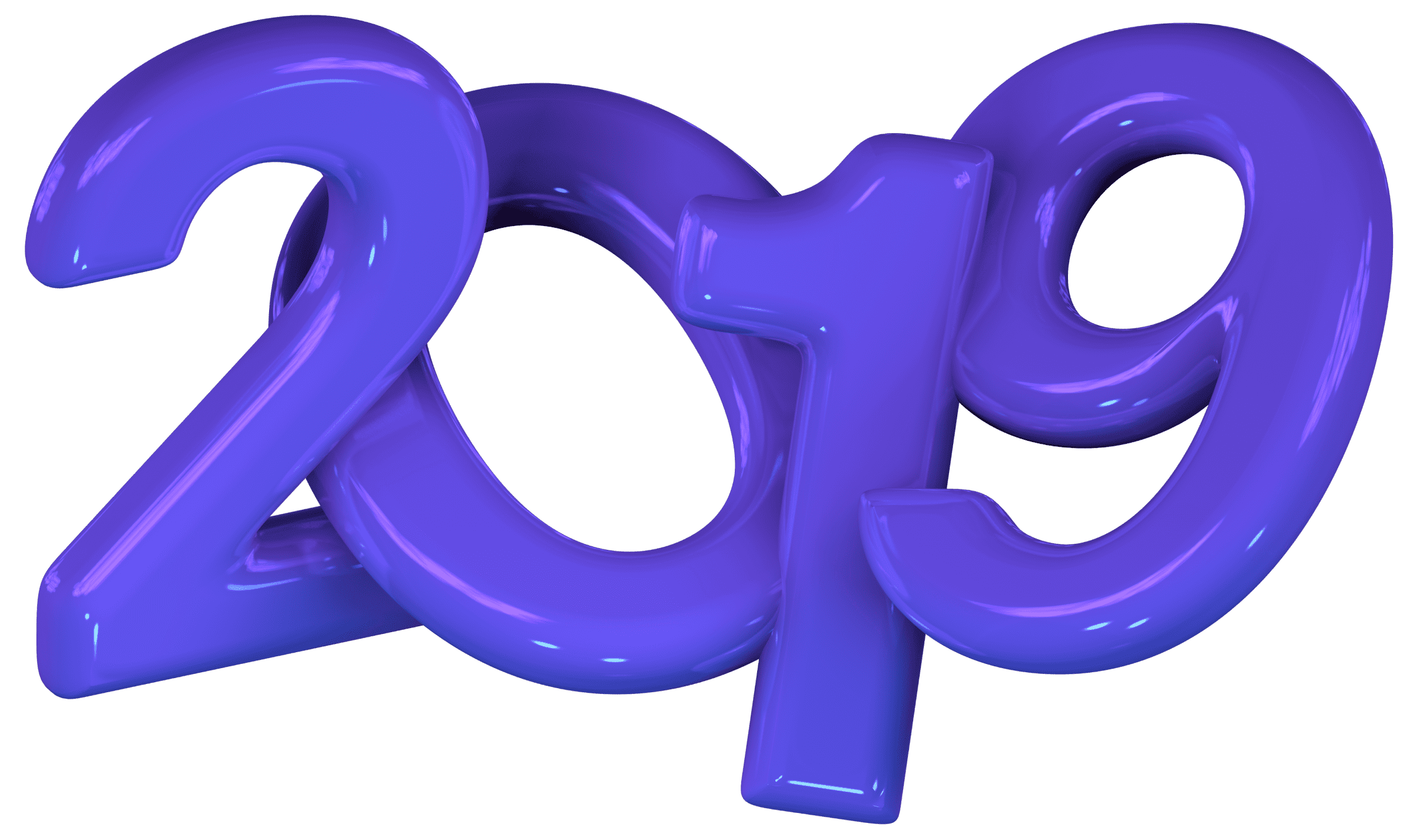
We took the first steps in exploring Kotlin for Data Science
conda install kotlin-jupyter-kernel -c jetbrains
Android development became
Kotlin-first!
We kicked off Kotlin/Everywhere
A series of community-led events co-organized by JetBrains and Google.
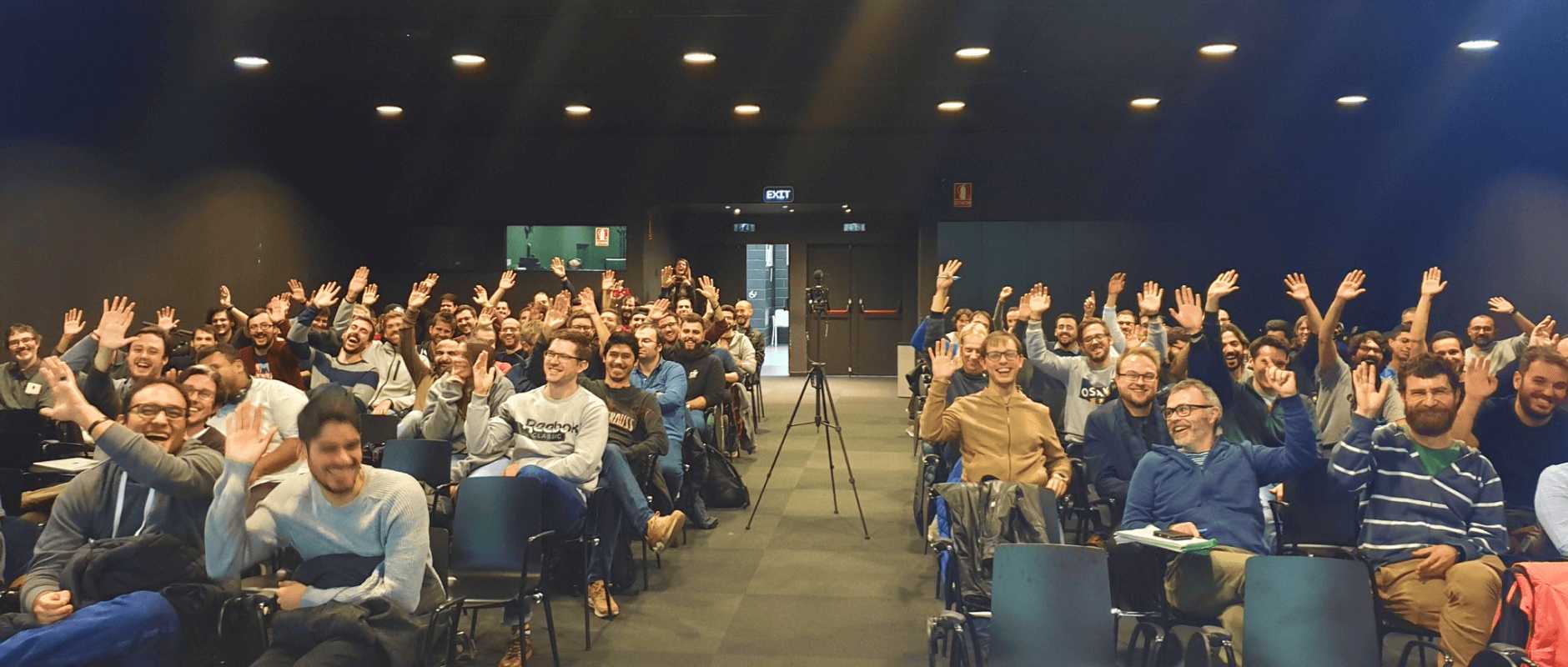
We implemented SAM conversions for Kotlin classes
fun interface Action {
fun run()
}
fun runAction(a: Action) = a.run()
fun main() {
runAction {
println("Hello, KotlinConf!")
}
}
The first ever Kotlin Heroes contest took place on Codeforces
KotlinConf 2019 was held in Copenhagen
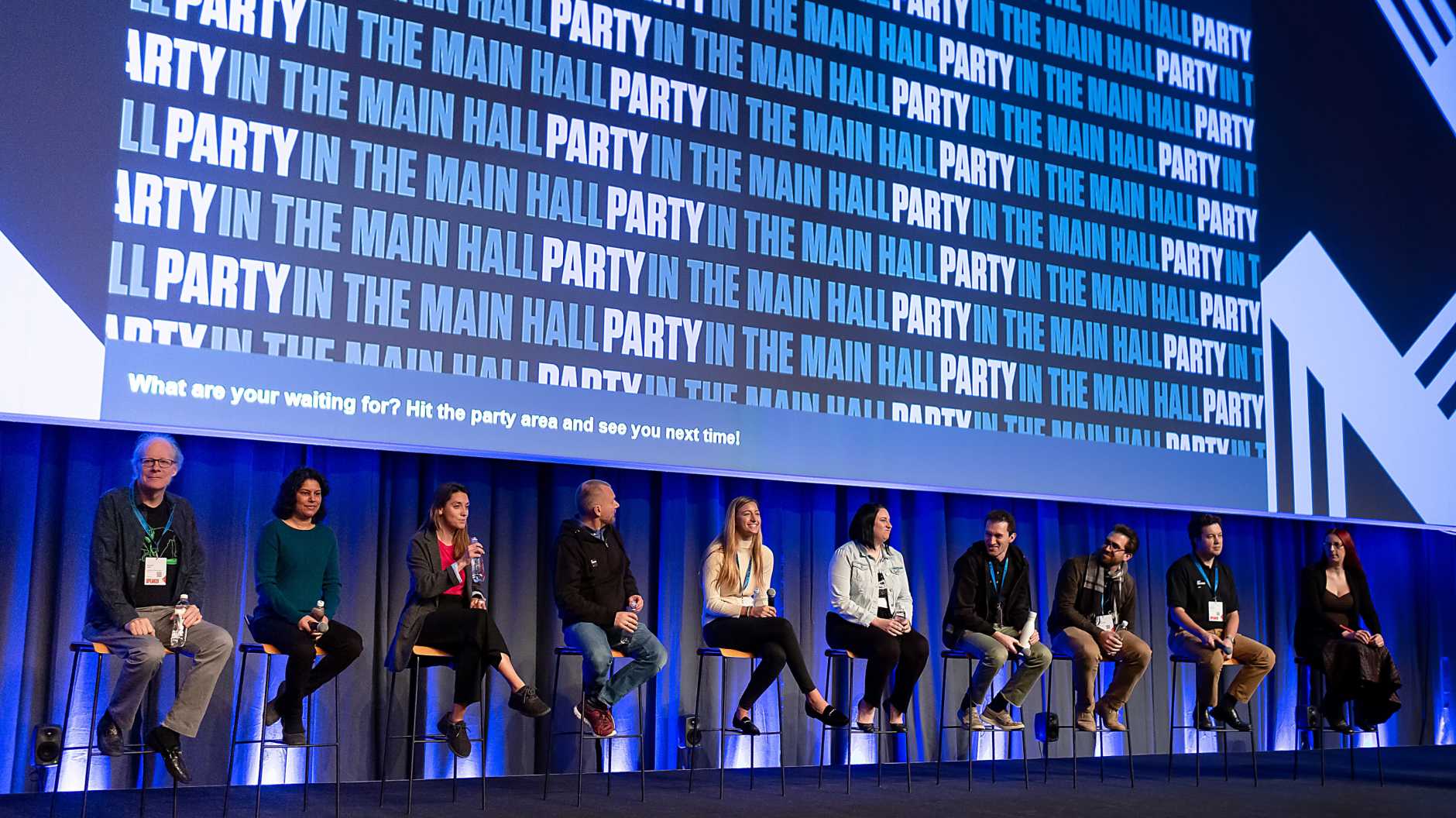
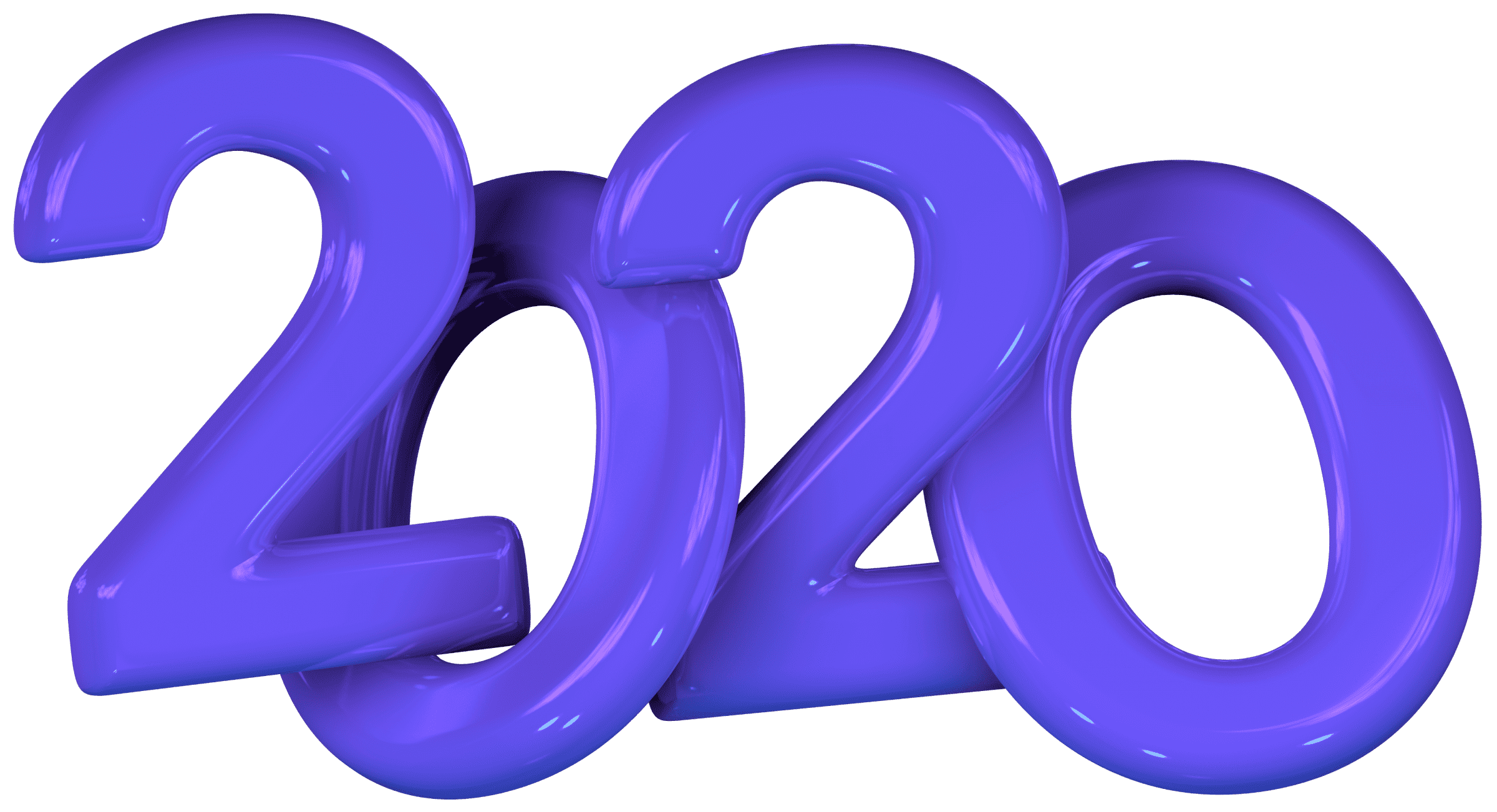
Kotlin Multiplatform Mobile moved to Alpha
This signified the Kotlin team’s full commitment to improving this technology and helping it evolve. It was also a signal that the product would develop quickly.
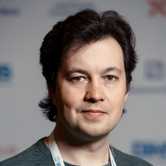
Roman Elizarov became the new Project Lead for Kotlin, after Andrey Breslav stepped down
kotlinx.serialization 1.0 was released
import kotlinx.serialization.*
import kotlinx.serialization.json.*
@Serializable
data class User(val name: String, val yearOfBirth: Int)
fun main() {
// Serialization (Kotlin object to JSON string)
val data = User("Louis", 1901)
val string = Json.encodeToString(data)
println(string) // {"name":"Louis","yearOfBirth":1901}
// Deserialization (JSON string to Kotlin object)
val obj = Json.decodeFromString<User>(string)
println(obj) // User(name=Louis, yearOfBirth=1901)
}
A new release cadence was announced for Kotlin and the Kotlin plugin
Kotlin 1.X will be released every six months.
The “Atomic Kotlin” book was published
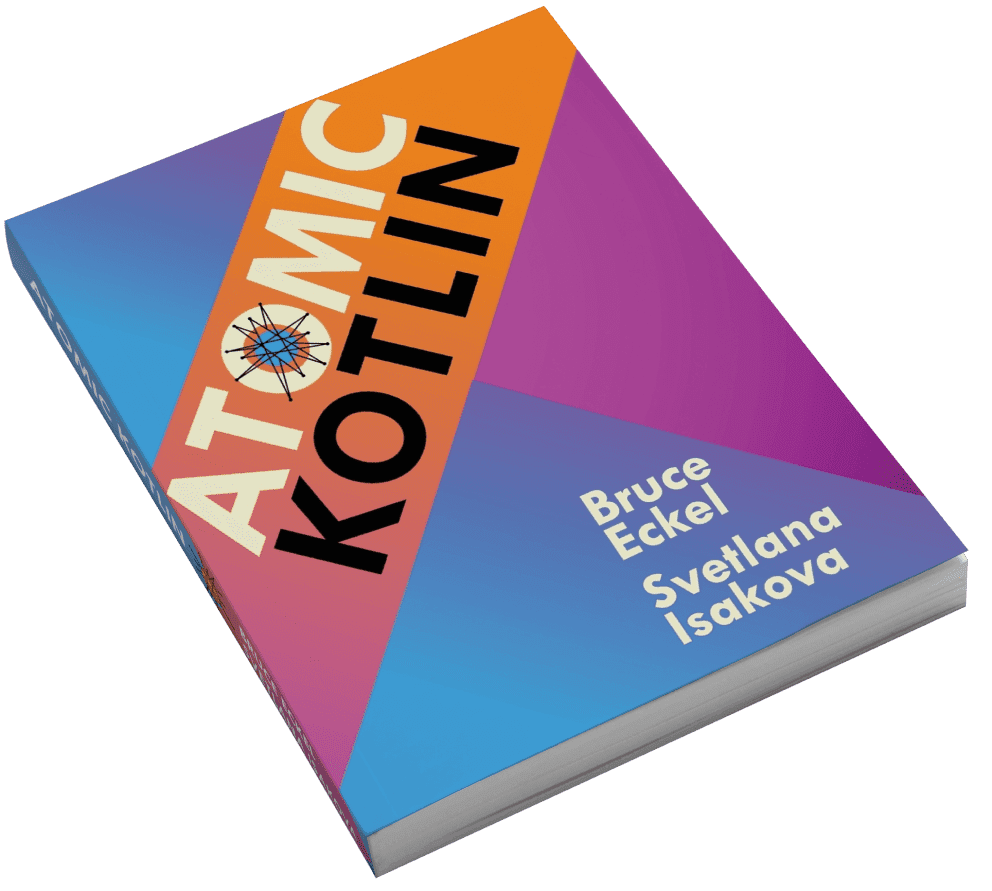
What about you?
Try Kotlin now →
or share your #10yearsofkotlin
story and win a T-shirt